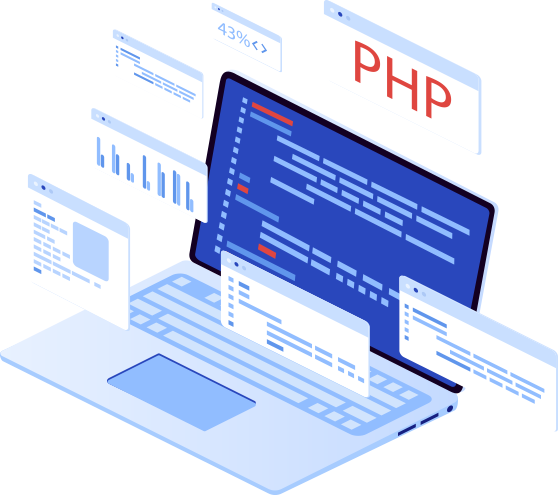
PHP Best Practices: Writing Clean and Efficient Code
PHP Introduction:
Commonly used in PHP web progress is Strips. Writing a clean Kudo, fixing, safety, and employment are all necessary to follow the greatest things to read, scatter, and seize, regardless of how short you are or what you are doing for work or a company.
We’ll look at important Php best practices in this post, such as maintainability, performance optimization, security precautions, and code standards. You will have a firm grasp on how to develop code of superior quality by the end.
Table of Contents
1. Adhere to PHP Coding Guidelines
Coding standards guarantee consistency across teams and projects, which makes code easier to understand and maintain. The most widely used PHP code standards are the PHP Standards Recommendations (PSR), which were developed by the it-FIG (PHP Framework Interoperability Group).
1.1. Standards for PSR Coding
- PSR-1: Basic Coding Standard (for example, class names should be in Pascal Case, methods should be in camel Case, and constants should be in uppercase).
- PSR-2: Coding Style Guide (curly brackets on new lines, four spaces for indentation, etc.).
- PSR-4: Autoloading Standard (for effective class organization and aautoloading. PSR-1: Basic Coding Standard (for example, class names should be in Pascal Case, methods should be in camel Case, and constants should be in uppercase).
1.2. Customs of Naming
Use meaningful variable names:
$user Age = 25; // Good
$au = 25; // Bad
Follow proper function and class naming:
class UserManager {} // Good
class usermanager {} // Bad
1.3. Spacing and Indentation
Always use 4 spaces instead of tabs for indentation.
Properly space operators and keywords for readability:
if ($age > 18) {
echo "You are an adult.";
}
2. Employ Tight Typing
PHP is weakly typed by default, which may result in strange behavior. Strict typing regulations help to avoid type-related errors.
Enable strict types at the beginning of your PHP files:
declare(strict_types=1);
function addNumbers(int $a, int $b): int {
return $a + $b;
}
echo addNumbers(5, 10); // Output: 15
3. Protect Your PHP Code
When creating PHP apps, security is of utmost importance. The following are important security best practices:
3.1. Avoid Injection of SQL
Use prepared statements instead of concatenating user input in SQL queries:
$pdo = new PDO('mysql:host=localhost;dbname=test', 'root', '');
$stmt = $pdo->prepare("SELECT * FROM users WHERE email = :email");
$stmt->execute(['email' => $_POST['email']]);
$user = $stmt->fetch();
3.2. Clean User Data
Never trust user input. Use htmlspecialchars() to prevent XSS attacks:
$cleanInput = htmlspecialchars($_POST['username'], ENT_QUOTES, 'UTF-8');
3.3. Safely Store Passwords
Use password_hash() and password_verify() for password storage and verification:
$hashedPassword = password_hash('mypassword', PASSWORD_DEFAULT);
if (password_verify('mypassword', $hashedPassword)) {
echo "Password is valid!";
}
3.4. Turn Off Production Error Display
Errors can expose sensitive information. Hide them in production:
ini_set('display_errors', 0);
error_reporting(0);
4. Enhance Efficiency
Performance optimization guarantees the smooth operation and scalability of your PHP application.
4.1. Use Caching
- Leverage caching mechanisms like:
- Opcode caching with OPcache
- Query caching with Redis or Memcached
4.2. Optimize Database Queries
Use indexes for faster lookups.
Fetch only the required data:
SELECT id, name FROM users WHERE status = 'active';
4.3. Employ Effective Loops
Avoid unnecessary loops and optimize them:
foreach ($users as $user) {
echo $user['name'];
}
4.4. Minimize Memory Usage
unset($largeArray);
5. Write Code That Is Maintainable
Long-term effectiveness, simpler debugging, and teamwork are all guaranteed by maintainable code.
5.1. Apply OOP (Object-Oriented Programming).
class User {
private string $name;
public function construct(string $name) {
$this->name = $name;
}
public function getName(): string {
return $this->name;
}
}
$user = new User("John Doe");
echo $user->getName();
5.2. Follow the DRY Principle
class Logger {
public function log(string $message) {
echo $message;
}
}
class UserService {
private Logger $logger;
public function __construct(Logger $logger) {
$this->logger = $logger;
}
public function createUser() {
$this->logger->log("User created.");
}
}
$logger = new Logger();
$userService = new UserService($logger);
$userService->createUser();
6. Make use of GIT (version control).
6.1. Follow a Git Workflow
Use branches (feature-branch, bugfix-branch).
Write meaningful commit messages:
git commit -m “Fixed user authentication bug”
6.2. Use .gitignore for Sensitive Files
.env
vendor
node_modules
7. Write Unit Tests
use PHPUnit\Framework\TestCase;
class MathTest extends TestCase {
public function testAddition() {
$this->assertEquals(4, 2 + 2);
}
}
Run tests using:
phpunit tests/
8. Keep a Record of Your Code
/**
* Adds two numbers.
*
* @param int $a
* @param int $b
* @return int
*/
function add(int $a, int $b): int {
return $a + $b;
}